Yes, you read it right. Now you can use python script in the backend with the android studio to perform different operations. The advantage of using Python in the android studio is that you can use Python in different libraries such as OpenCV, Matplotlib, Numpy, Pandas and much more.
Why Python in Android Studio?
As you know, in Python, we have to write fewer lines of code, which is why Python is the most popular among all programming languages. So we will take this advantage in writing code to develop android applications.
Now Let’s see what is Chaquopy?
Chaquopy is the Python SDK for Android that provides you with a complete facility to calling Python code from Java or kotlin.
Chaquopy gives you the way to use Python’s most trending libraries like TensorFlow, SciPy with android studio.
So in this article, you will get complete information about –
- How to integrate Chaquopy in android studio.
- How to trigger Python script from Java code.
- How to install Python Library in android studio.
Chaquopy Setup
1. First of all, add the below dependencies in the project-level build.gradle file.
buildscript { repositories { ... maven { url "https://chaquo.com/maven" } } dependencies { ... classpath "com.chaquo.python:gradle:9.1.0" } }
2. Now add the below dependencies in the app-level build.gradle file.
apply plugin: 'com.chaquo.python' android { defaultConfig { python { buildPython "C:/Users/sarthak/AppData/Local/Programs/Python/Python39/python.exe" } ndk { abiFilters "x86", "armeabi-v7a" } sourceSets { main { python.srcDir "src/main/python" } } }
Note:- the above mentioned buildPython path is for my system, use your python.exe path to setup, abiFilters is used to enable ABI for mobile and emulator, and finally, will use srcDir to keep all python scripts that we will trigger from the android app.
3. Now create a Python file (myscript.py) in the folder mentioned in step 2 (src/main/python). For this example, you need to create a function in a Python file that we will call through our android java code. Check the below python code.
def main(): return “working”
So here, a simple method is added that will return a string, and that string we will be used to show as a Toast message in our android app.
4. Now call the above Python script from our Java code.
Python py = Python.getInstance(); PyObject pyobj = py.getModule("myscript"); PyObject obj = pyobj.callAttr("main"); Toast.makeText(this, obj.toString(), Toast.LENGTH_SHORT).show();
In the above code first we created Python Instance, then we are calling our python script (myscript) using getModule() function, then we are calling main function that we added in python script and storing its output in ‘obj’ Object and finally, we are showing Toast message using our object.
So in this way we are triggering Python script in android studio.
5. Now, if you want to add any Python library in chaquopy, then use the below piece of code in defaultConfig (check step 2)
python{ pip{ install "numpy" } }
6. Finally build.gradle file code will be like this
apply plugin: 'com.android.application' apply plugin: 'com.chaquo.python' android { compileSdkVersion 30 buildToolsVersion "30.0.1" defaultConfig { applicationId "com.andro.andro01.chaquopylatestversion" minSdkVersion 16 targetSdkVersion 30 versionCode 1 versionName "1.0" testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner" ndk { abiFilters "x86" } python { buildPython "C:/Users/sarthak/AppData/Local/Programs/Python/Python39/python.exe" } sourceSets { main { python.srcDir "src/main/python" } } python{ pip{ install "numpy" } } } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro' } } } dependencies { implementation fileTree(dir: "libs", include: ["*.jar"]) implementation 'androidx.appcompat:appcompat:1.2.0' implementation 'androidx.constraintlayout:constraintlayout:2.0.4' testImplementation 'junit:junit:4.13.1' androidTestImplementation 'androidx.test.ext:junit:1.1.2' androidTestImplementation 'androidx.test.espresso:espresso-core:3.3.0' }
Final Output:
Note :- You can download full source code of chaquopy setup from our “Source Code” section.
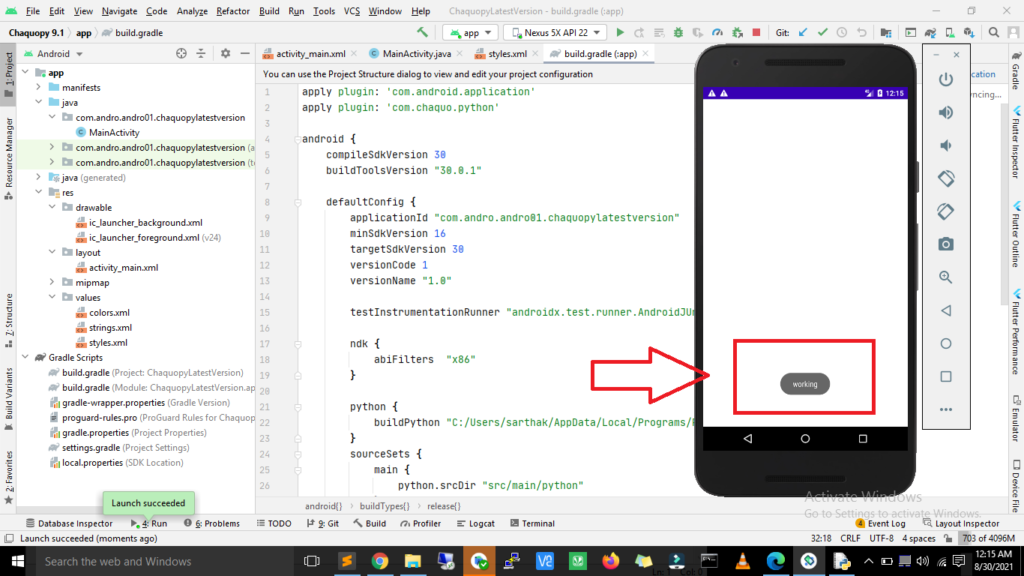
So in this way, you can run python scripts in android and install any python library.
If you have any questions, then you can comment below or message us on Instagram for quick response.
Check out how to make music player using python kivy.
Pingback: How to Add Firebase in Android Studio - ProgrammingFever